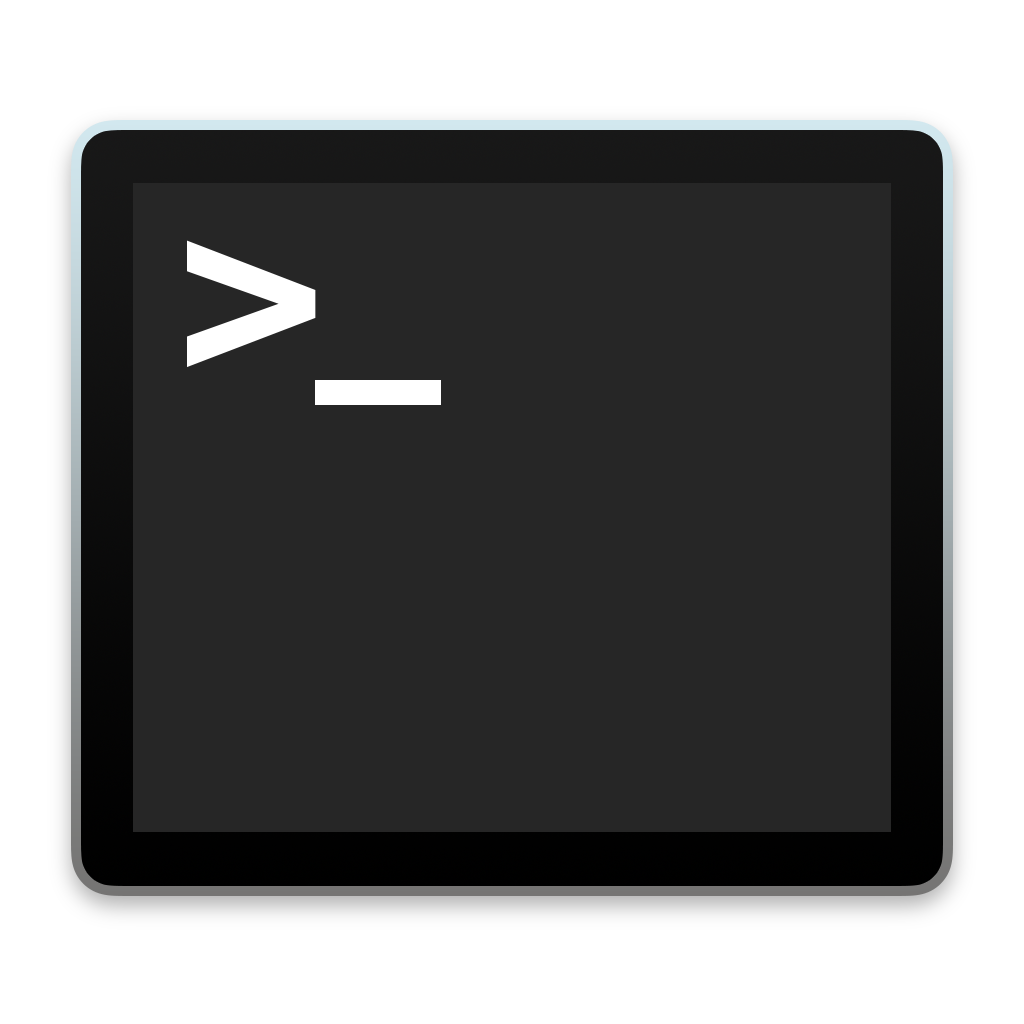
$
. In the examples on this page we'll just use that symbol (without any other text) to represent the prompt:
$
Commands are entered at the prompt. You don't need to click; just start typing. Don't type the $
; that should already be there for you. Only enter the text that comes after the $
prompt.
A typical command is run by giving the name of the command, followed by any arguments. Pressing the return key executes the command.
For example, the echo command prints all of its remaining arguments to the terminal. Note that there is no prompt on the line with the output. Try this command out in your terminal window to ensure it looks the same.
$ echo These are the arguments to echo These are the arguments to echo $
You'll need to make a directory (folder) to store this program. Think about what you want to name it, and which folder it should go in.
For example, suppose we want to call our new folder Hello World
, and we'd like to put it in a folder called APCS. The APCS folder already exists and is inside the Documents folder.
We can do this by running the command mkdir ("make directory") command, with the full path of directories as it's arguments (we have to put this in "quotes" because any spaces in the name will be interpreted as two arguments).
In the example, you'll see that we build the path from left to right with slashes (/
) between each directory; the $HOME represents your "home" folder on the computer (where all your files are). The next folder inside that is Documents, followed by APCS, and the last item is the one we want to create: Hello World.
If you run the command and nothing gets printed out, then it completed sucessfully. If you get a message back, the command did not complete. Make sure you've created any intermediate folders that the new folder is going into.
$ mkdir "$HOME/Documents/APCS/Hello World" $
We now need to "move into" this directory. On the command line, you don't open folders by double-clicking; instead you change the working directory and all future commands are interpreted within that context.
The cd ("change directory") does this. It takes the directory to move into as its argument. In this case, it's the exact same path that you created above, so you can select the text you wrote to create the directory, copy it, and paste it in after the cd command.
When you're done, you can confirm you're in the right place by runing the pwd ("print working directory") command. It should output the path that you're currently working in, which should end with the name of the directory you just created.
(In this case, the text at the beginning might not match as it will depend on the account name you're using on your computer. However, the final part should match the name of the directory you created.)
$ cd "$HOME/Documents/APCS/Hello World" $ pwd /Users/joebogus/Documents/APCS/Hello World $
At this point, we're ready to create our program, as we have a place to put it! We're going to create a file named HelloWorld.java inside this directory. You have two choices: edit it using the editor of your choice, or paste in the Java code on the command line.
If you have an editor, open it up and create a new file. Copy and paste the sample code into the file and save it. When you're prompted for the location to save it in, navigate to your new folder and save the file with the name HelloWorld.java.
If you don't have an editor to use, you can highlight the code shown, copy it, and then run the following command:
pbpaste > HelloWorld.java
That pastes the selected text and saves it into a new file named HelloWorld.java all in one step.
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello World!"); } }
To confirm that the file was created correctly, run the cat command, which reads a file and outputs it to the terminal. You should see the sample code that you entered in the previous step.
$ cat HelloWorld.java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello World!"); } } $
We're now ready to compile the program from source code into a binary form. The javac ("java compiler") program does this, using the name of the source code file as its argument.
If there are any errors, they will be printed on the terminal. You must fix any errors, save the file, and try again.
Once the compilation is complete, you may use the ls ("list") command to show the files in the directory. There should be a new file named HelloWorld.class that was created by the compiler.
$ javac HelloWorld.java $ ls HelloWorld.class HelloWorld.java $
With the program compiled, it's ready to execute! The java command starts the Java Virtual Machine and executes the program starting with the given Java class name. Note that you do not need the .class extension; just the name of the class itself.
When run, the text from the System.out.println
in the program should appear on the terminal.
$ java HelloWorld Hello World! $
Congratulations! You've created, compiled, and run a Java program on the command line.
If you want to make changes to the program, you need to edit the HelloWorld.java source code file, save those change, run javac again, and then run java again. Try making a small change and recompiling your program.
$ sed -i '' 's/Hello World/Hello Someplace Else/g' HelloWorld.java $ javac HelloWorld.java $ java HelloWorld Hello Someplace Else! $
Knowing What You Don't Know
This example shows the complete process for a very simple program. However, it would not work well for anything more complex. For example:
- You normally should include a package declaration for all classes that you write. When you do so, the source code files must go in a directory structure that matches the package structure. Unfortunately, this makes "finding" the source code files a little trickier when you run javac.
- Compiling multiple source code files at once -- and producing multiple binary class files as a result -- tends to clutter up the working directory. Typically you would output your class files to a dedicated directory so they can be easily accessed or cleaned.
- Many programs rely on external libraries to provide some classes used in the program. We haven't covered how to load those libraries when compiling or running the program. We also haven't discussed Java's notion of a
CLASSPATH
to search for libraries.
Most complex projects us a build tool to automate the compilation and execution of their code so they don't need to keep track of each file and its exact location. Tools like Maven, Gradle, and Ant can do this, and many IDEs come with support to use these tools when developing Java software.